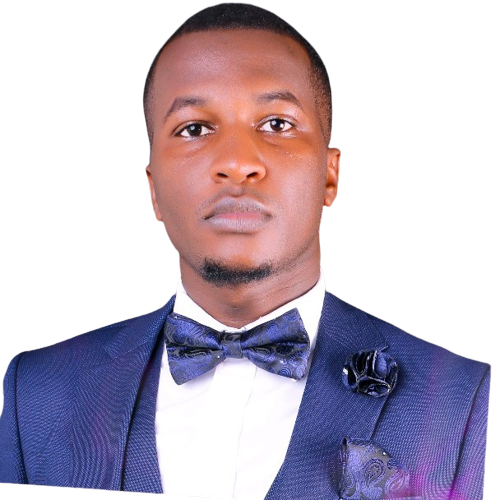
mmuthigani
Published on 05/30/2024
In PHP, loops are used to execute the same block of code multiple times. This can be useful for iterating over arrays or repeating a certain operation a specific number of times. PHP supports several types of loops, including for loops, while loops, and do-while loops.
Here is an example of a for loop in PHP:
for ($i = 0; $i < 10; $i++) {
// code to be executed
}
This for loop will execute the code inside the curly braces 10 times. On each iteration, the value of the variable $i
is increased by 1.
A while loop is another type of loop in PHP. It continues to execute the code inside the loop as long as a certain condition is true. Here is an example of a while loop:
$i = 0;
while ($i < 10) {
// code to be executed
$i++;
}
This while loop will execute the code inside the loop as long as the value of $i
is less than 10. The value of $i
is increased by 1 at the end of each iteration to prevent the loop from running indefinitely.
A do-while loop is similar to a while loop, but it always executes the code inside the loop at least once. Here is an example of a do-while loop:
$i = 0;
do {
// code to be executed
$i++;
} while ($i < 10);
This do-while loop will execute the code inside the loop at least once, and then it will continue to execute the code as long as the value of $i
is less than 10.
In PHP, loops can be used to perform a variety of tasks, such as iterating over arrays to process data, or repeating an operation a certain number of times.
mmuthigani
Published on 05/30/2024
mmuthigani
Published on 02/10/2024
mmuthigani
Published on 01/12/2024